Easy-Appointments is a powerful and flexible WordPress plugin designed to streamline appointment scheduling for businesses and professionals. It enables users to manage appointments, schedule services, and handle customer bookings seamlessly.
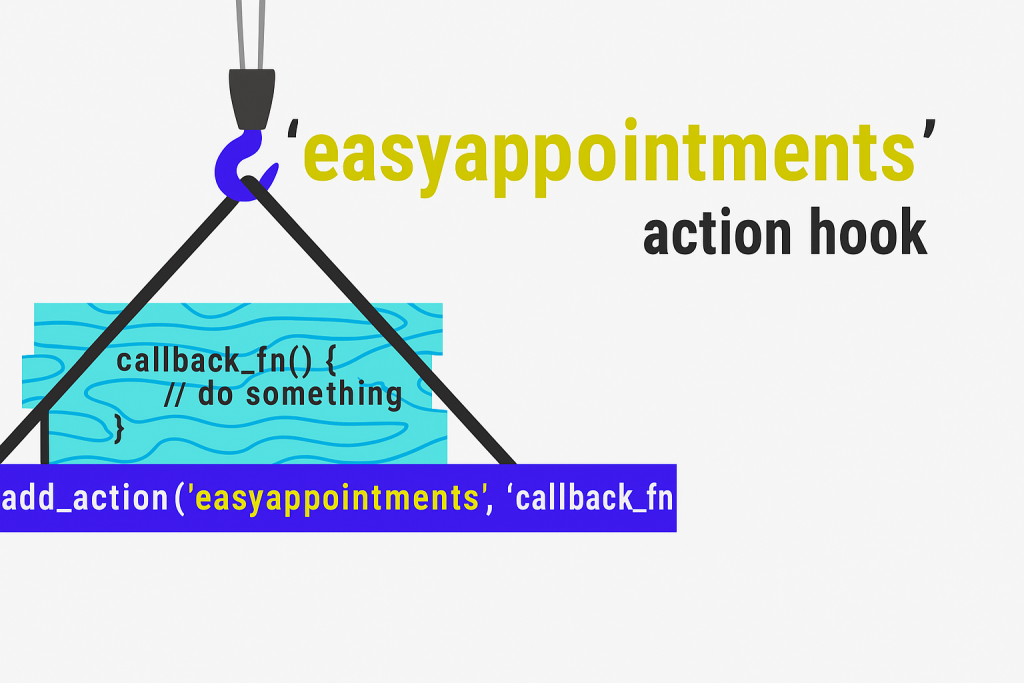
However, every business has unique requirements, and sometimes, the default functionality of Easy-Appointments may not be enough. To address this, the plugin provides various hooks and callbacks that allow developers to customize and extend its features without modifying the core plugin files.
What Are Hooks and Callbacks? #
Hooks in WordPress allow developers to modify or extend the functionality of a plugin without altering its core code. Hooks are divided into two types:
- Actions – These are triggered at specific events, allowing custom code to run when those events occur.
- Filters – These modify data before it is processed, enabling developers to customize how the plugin behaves.
Callbacks refer to custom functions that execute when a specific event occurs, providing a way to integrate new features into the existing system.
Uses of Hooks and Callbacks #
Frontend Callbacks #
Frontend callbacks allow you to integrate custom JavaScript to modify the user experience during appointment booking.
On Creating an Appointment #
- Event:
easyappnewappointment
(Customer creates an appointment) - Example: You can trigger a Google Analytics event when an appointment is created.
// example
document.addEventListener( 'easyappnewappointment', function( event ) {
// place here code that will be executed when customer book appointment
// ga( 'send', 'event', 'EasyAppointments', 'submit' );
}, false );
On Selecting a Time Slot #
- Event:
easyappslotselects
- Example: Redirect users after selecting a time slot.
// example
document.addEventListener( 'easyappslotselects', function( event ) {
// redirect
// event.details holds information on location, service, worker, price, time and date since EA version 3.7.2
window.location.replace("https://wordpress.org/plugins/easy-appointments/");
}, false );
Note: This script should be placed in the JavaScript section of the relevant page.
Backend Callbacks(Ajax backend) #
Backend hooks allow developers to process additional data when a customer books an appointment or interacts with email actions.
On Booking an Appointment #
- Action:
ea_new_app_from_customer
- Example: When customer book appointment that can be used for additional data processing.
add_action('ea_new_app_from_customer', 'custom_callback_function', 10, 2);
function custom_callback_function($appointment_id, $appointment_data) {
// Custom processing
}
Email action redirect URL #
- Filters:
ea_confirmed_redirect_url
,ea_cancel_redirect_url
- Example: Redirect users to a custom page after confirming or canceling an appointment.(This custom filter allows you add custom redirect page instead of home page that is default. You must provide valid URL address.)
add_filter( 'ea_confirmed_redirect_url', 'custom_confirm_callback_function', 10, 1 );
function custom_confirm_callback_function( $currentUrl) {
// do stuff here
return '';
}
add_filter( 'ea_cancel_redirect_url', 'custom_cancel_callback_function', 10, 1 );
function custom_cancel_callback_function( $currentUrl) {
// do stuff here
return '';
}
Note: Do not edit the plugin files directly; instead, use a custom plugin or child theme.
Custom Fields or Alter Custom Fields filter #
- Filter:
ea_form_rows
- Example: Modify custom fields in the booking form.
add_filter('ea_form_rows', 'alter_custom_fields');
function alter_custom_field($fields) {
// do stuff here
return $fields;
}
Filter For Controlling User Capabilities for Menu Items #
- Filter:
easy-appointments-user-menu-capabilities
- Example: Restrict access to specific menu items based on user role.
There is filter called when adding menu item in admin part along with menu items you need to allow particular user to make calls.
add_filter('easy-appointments-user-menu-capabilities', 'custom_capabilities', 10, 2);
function custom_capabilities($default_capability, $menu_slug) {
// do stuff here depending on user that is logged in
return $default_capability;
}
Managing User Access to Ajax Requests #
- Filter:
easy-appointments-user-ajax-capabilities
- Example: Control access to AJAX-based settings.
In order to do that there is additional filter to use. Default capability is manage_options
add_filter('easy-appointments-user-ajax-capabilities', 'admin_ajax_capabilities', 10, 2);
function admin_ajax_capabilities($default_capability, $ajax_resource) {
// do stuff here depending on user that is logged in
// $arax_resource options are ['settings','services','locations','workers','connections', 'tools', 'reports']
return $default_capability;
}
Best Practices for Customization #
- Avoid editing the EasyAppointments plugin files directly. Instead, use a custom plugin or a child theme to store your modifications.
- Use the provided hooks and filters to ensure compatibility with future plugin updates.
- Test changes in a staging environment before applying them to a live website.